Creating a smooth integration with Verfacto CRM is as easy as it gets, thanks to our API. This simplified guide will lead you through the basic steps to begin your development journey using the Verfacto API.
1. Authentication
How to get your API key
To begin your Verfacto API journey, you’ll need to obtain a unique API key. To do this, go to your Verfacto account settings, find the integrations section, and simply generate your personalized API key.
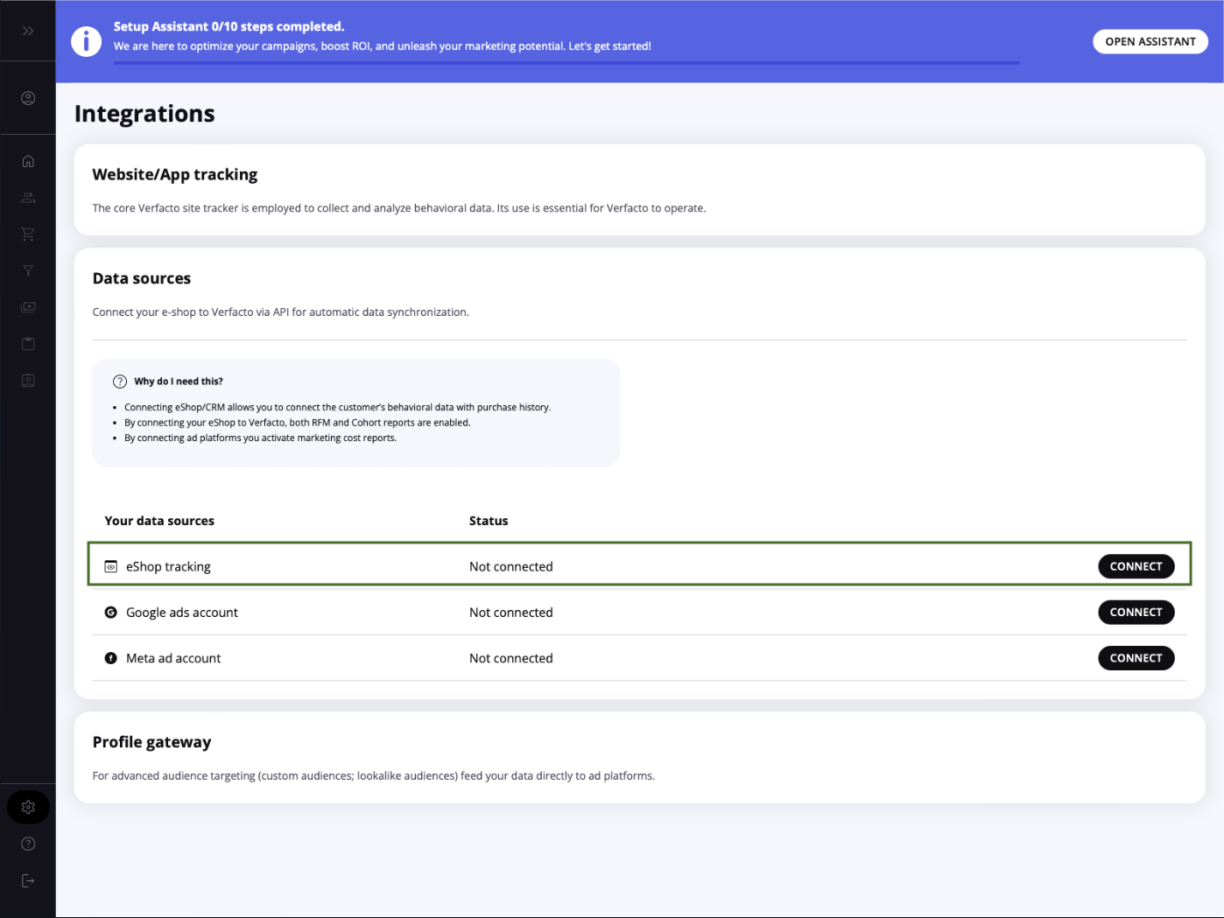
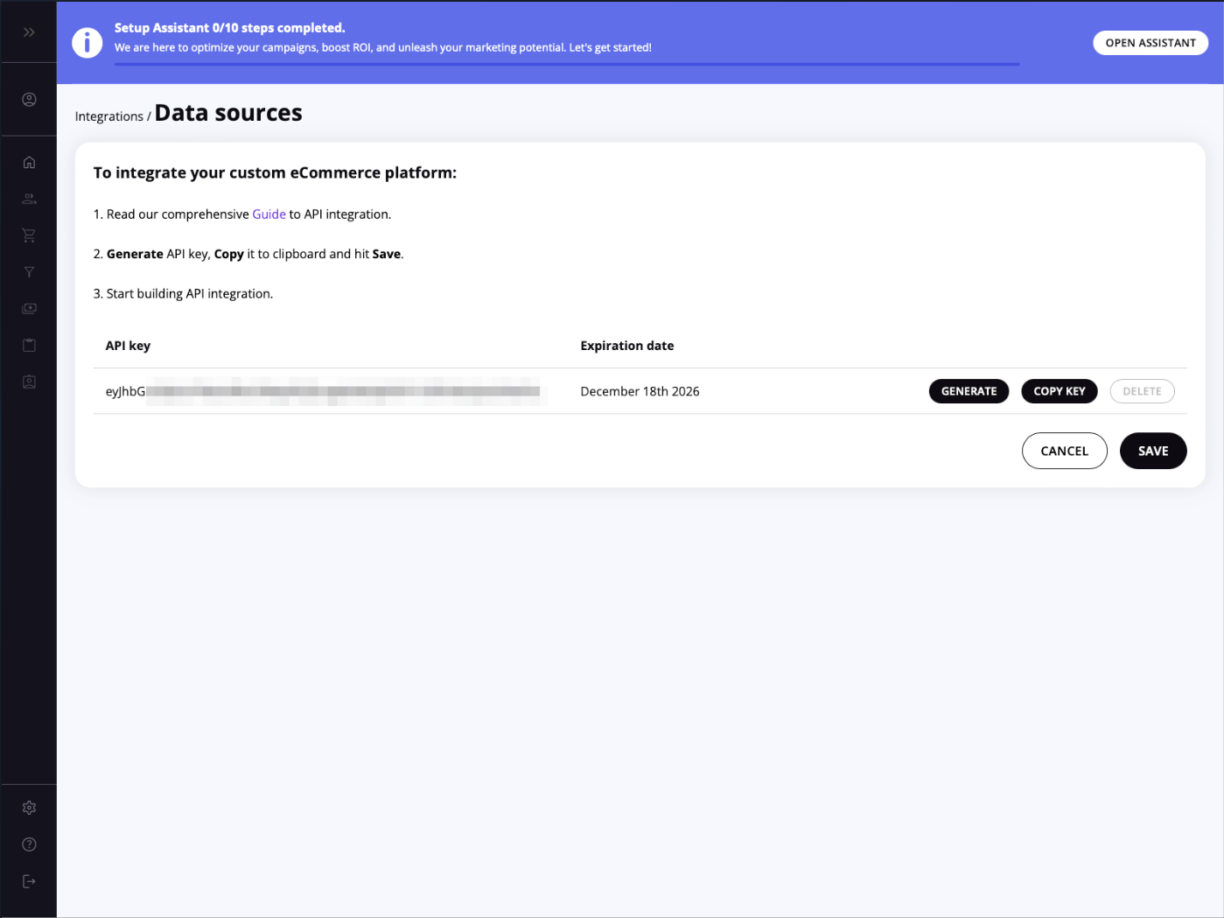
Include your API key in requests
Ensuring the security of your requests is crucial. Simply add your API key in the header of each request under the ‘Authorization’ field.
GET /crm/v1/customers
Host: api.verfacto.com
Authorization: Bearer YOUR_API_KEY
2. Making your first request
Understanding the base URL
You can access the Verfacto API through the base URL:
https://api.verfacto.com/crm/v1
Navigating endpoint structures
Endpoints follow a consistent structure:
https://api.verfacto.com/crm/v1/{endpoint}
Using pagination
List endpoints support pagination:
https://api.verfacto.com/crm/v1/{endpoint}?offset=0&limit=20
A sample request in action
Let’s kick things off with a simple request using customer data:
GET /crm/v1/customers
Host: api.verfacto.com
Authorization: Bearer YOUR_API_KEY
3. Handling responses
Decoding HTTP status codes
Responses communicated through standard HTTP status codes.
A successful request typically returns 200 OK.
Embracing the JSON response format
Responses are neatly packaged in JSON format.
Ensure your application is equipped to handle JSON responses.
4. Exploring endpoints with real-world examples
Example 1 – Creating the customer profile
Request
POST /crm/v1/customers
Host: api.verfacto.com
Authorization: Bearer YOUR_API_KEY
Content-Type: application/json
{
"shop_customer_id": "33665599", // customer identifier within your platform
"email": "[email protected]",
"phone": "+98272723",
"gender": "male",
"birthdate": "2003-01-02 04:05:06", // birth date of a customer
"created": "2023-01-02 04:05:06", // date when customer was created in our platform
"updated": "2023-01-03 04:05:06", //last time when customer entry was changed
}
Response
{
"id": 575859
"shop_customer_id": "33665599"
"birthdate": "2003-01-02T00:00:00.000Z",
"created": "2023-01-02T04:05:06.000Z",
"email": "[email protected]",
"gender": "male",
"phone": "+98272723",
"updated": "2023-01-03T04:05:06.000Z"
}
Example 2 – Listing created customers
Request
GET /crm/v1/customers?offset=0&limit=10
Host: api.verfacto.com
Authorization: Bearer YOUR_API_KEY
Content-Type: application/json
Response
[
{
"id": 575859
"shop_customer_id": "33665599"
"birthdate": "2003-01-02T00:00:00.000Z",
"created": "2023-01-02T04:05:06.000Z",
"email": "[email protected]",
"gender": "male",
"phone": "+98272723",
"updated": "2023-01-03T04:05:06.000Z"
}
]
Example 3 – Creating shop products
Request
POST /crm/v1/products
Host: api.verfacto.com
Authorization: Bearer YOUR_API_KEY
Content-Type: application/json
{
"shop_product_id": "p-3333-0110",
"name": "Very popular product No1",
"sku": "p-3333",
"category": "Most wanted products",
"created": "2023-02-01T10:11:22Z",
"subcategory": "Summer",
"updated": "2023-02-02T14:14:14Z"
}
Response
{
"id": 115588,
"shop_product_id": "p-3333-0110",
"name": "Very popular product No1",
"sku": "p-3333",
"category": "Most wanted products",
"created": "2023-02-01T10:11:22Z",
"subcategory": "Summer",
"updated": "2023-02-02T14:14:14Z"
}
Request
POST /crm/v1/products
Host: api.verfacto.com
Authorization: Bearer YOUR_API_KEY
Content-Type: application/json
{
"shop_product_id": "p-3334-0110",
"name": "Very popular product No2",
"sku": "p-3334",
"category": "Most wanted products",
"created": "2023-02-01T11:11:22Z",
"subcategory": "Winter",
"updated": "2023-02-02T15:15:15Z"
}
Response
{
"id": 115589,
"shop_product_id": "p-3334-0110",
"name": "Very popular product No2",
"sku": "p-3334",
"category": "Most wanted products",
"created": "2023-02-01T11:11:22Z",
"subcategory": "Winter",
"updated": "2023-02-02T15:15:15Z"
}
Example 4 – Creating order for the customer
Request
POST /crm/v1/orders
Host: api.verfacto.com
Authorization: Bearer YOUR_API_KEY
Content-Type: application/json
{
"shop_customer_id": "33665599",
"shop_order_id": "g-32556-54215-ggsd44-544",
"order_number": "#544",
"status": "payment pending",
"total_discount": 0,
"total_price": 100.22,
"currency": "EUR",
"purchased_at": "2023-08-24T14:15:22Z",
"created": "2023-08-24T14:15:22Z"
}
Response
{
"id": 272829,
"shop_customer_id": "33665599",
"shop_order_id": "g-32556-54215-ggsd44-544"
"order_number": "#544",
"status": "payment pending",
"total_discount": 0,
"total_price": 100.22,
"currency": "EUR",
"purchased_at": "2023-08-24T14:15:22Z",
"created": "2023-08-24T14:15:22.000Z",
"updated": "2023-08-24T14:15:22.000Z"
}
Example 5 – Adding line items for the order based on internal order ID
Request
POST /crm/v1/orders/272829/line_items
Host: api.verfacto.com
Authorization: Bearer YOUR_API_KEY
Content-Type: application/json
{
"shop_order_id": "g-32556-54215-ggsd44-544",
"line_item_id": "ln33669945",
"product_id": "p-3333-0110",
"product_name": "Very popular product No1",
"quantity": 1,
"price": 100.22,
"discount": 0,
"currency": "EUR",
"sku": "p-3333",
"created": "2023-08-24T14:15:22Z"
}
Response
{
"id": 414243,
"shop_order_id": "g-32556-54215-ggsd44-544",
"line_item_id": "ln33669945",
"product_id": "p-3333-0110",
"product_name": "Very popular product No1",
"quantity": 1,
"price": 100.22,
"discount": 0,
"currency": "EUR",
"sku": "p-3333",
"created": "2023-08-24T14:15:22Z"
}
Request
POST /crm/v1/orders/272829/line_items
Host: api.verfacto.com
Authorization: Bearer YOUR_API_KEY
Content-Type: application/json
{
"shop_order_id": "g-32556-54215-ggsd44-544",
"line_item_id": "ln33669946",
"product_id": "p-3334-0110",
"product_name": "Very popular product No2",
"quantity": 2,
"price": 33.44,
"discount": 0,
"currency": "EUR",
"sku": "p-3334",
"created": "2023-08-25T15:15:22Z"
}
Response
{
"id": 414244,
"shop_order_id": "g-32556-54215-ggsd44-544",
"line_item_id": "ln33669946",
"product_id": "p-3334-0110",
"product_name": "Very popular product No2",
"quantity": 2,
"price": 33.44,
"discount": 0,
"currency": "EUR",
"sku": "p-3334",
"created": "2023-08-25T15:15:22Z"
}
Example 6 – Viewing created line items
Request
GET /crm/v1/orders/272829/line_items
Host: api.verfacto.com
Authorization: Bearer YOUR_API_KEY
Content-Type: application/json
Response
[
{
"id": 414243,
"shop_order_id": "g-32556-54215-ggsd44-544",
"line_item_id": "ln33669945",
"product_id": "p-3333",
"product_name": "Very popular product No1",
"quantity": 1,
"price": 100.22,
"discount": 0,
"currency": "EUR",
"sku": "p-3333",
"created": "2023-08-24T14:15:22Z"
},
{
"id": 414244,
"shop_order_id": "g-32556-54215-ggsd44-544",
"line_item_id": "ln33669946",
"product_id": "p-3334",
"product_name": "Very popular product No2",
"quantity": 2,
"price": 33.44,
"discount": 0,
"currency": "EUR",
"sku": "p-3334",
"created": "2023-08-25T15:15:22Z"
}
]
Example 7 – Removing a line item from the order
Request
DELETE /crm/v1/orders/272829/line_items/414143
Host: api.verfacto.com
Authorization: Bearer YOUR_API_KEY
Content-Type: application/jso
Example 8 – Completing the order
Request
POST /crm/v1/orders/272829
Host: api.verfacto.com
Authorization: Bearer YOUR_API_KEY
Content-Type: application/json
{
"shop_customer_id": "33665599",
"shop_order_id": "g-32556-54215-ggsd44-544"
"order_number": "#544",
"status": "payment pending",
"total_discount": 0,
"total_price": 100.22,
"created": "2023-08-24T14:15:22.000Z",
"updated": "2023-08-24T14:15:22.000Z"
}
Response
{
"id": 272829,
"shop_customer_id": "33665599",
"shop_order_id": "g-32556-54215-ggsd44-544"
"order_number": "#544",
"status": "completed",
"total_discount": 0,
"total_price": 100.22,
"currency": "EUR",
"purchased_at": "2023-08-24T14:15:22Z",
"created": "2023-08-24T14:15:22.000Z",
"updated": "2023-08-24T14:15:22.000Z"
}
5. Error handling made easy
Common error codes
400 Bad Request
: Malformed request.401 Unauthorized
: Invalid API key.404 Not Found
: Endpoint not found.500 Internal Server Error
: Server issues.
Troubleshooting tips for a smooth experience
Check the error message in the response insights when troubleshooting an issue.
6. Additional resources for your journey
A closer look at the API reference
For more intricate details on specific endpoints and parameters, consult our API Reference.
Support and contact information for your queries
Do you have questions or need assistance? Reach out to our support team at [email protected].
Congratulations, you’ve now mastered the basics of integrating Verfacto CRM into your applications using our API.
Refer to our API Reference for more detailed information, or reach out to our support team if you need further assistance.